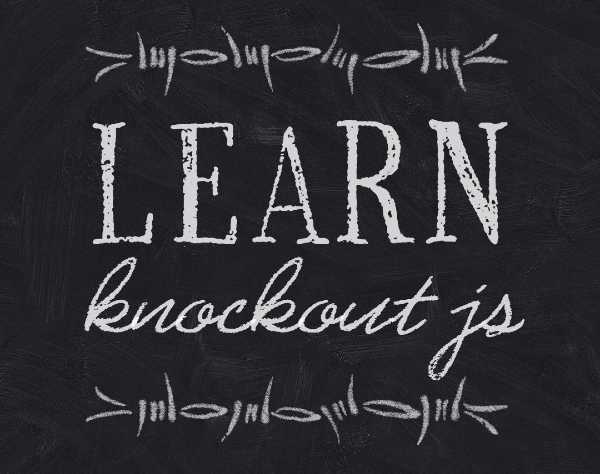
What is Knockout.js and why is it a very popular Javascript library?
-
Uses the Model-View-View Model pattern to help create dynamic/automatic UI refreshes
-
Dynamic class bindings with observable objects in your script
-
Works seamlessly with ASP.NET MVC Razor Views
-
Knockout.js is different from jQuery because it focuses on creating dynamic, single web page applications
-
Bind all aspects of a DOM element using the data bind attribute
-
Whether you are a beginner or expert with Knockout, these tutorials will provide you with essential examples by providing real-world demos.
Data bind attribute Function
I mentioned earlier that in these knockout js tutorial that your code will rely heavily on the data bind property in your web application. This is handled with the MVVM pattern by using html to data bind your Javascript object to your script functions. This script can often be used in a span tag.
Observable array Function
Your view model (which I typically instantiate with var self = this) will probably have a lot of data linked to your server side code in your HTML code. Before diving into code relating to the observableArray I also have created a great list of articles you can visit on Javascript arrays. This is a great place for beginners to learn logic to explain and search the details of managing the data within your observable arrays. The code on this website has great comments and is a good template to add to your view model with events, comments, and query and get support with visible sample in your script tag. On occasion you may get errors like How to check for undefined in JavaScript so hopefully this will help.
Knockout.js Script Tag Examples
Below are a list of Knockout.js script articles that will provide the essential tools that span from beginner to an expert with Knockout.js tutorial by providing clear and concise demos with practical, real-world examples that provide value and many template html, script and form to watch for with the details created with input type, search, and any other function created. Whether you are looking for beginner Knockout.js tutorials or advanced tutorials these examples will log the essentials to become more proficient with the Knockout.js library script. If you are looking for something specific you can try a search on the html for a specific script tag or function that may span in form of a script default.
These Knockout tutorial span many function including integrating your script with an api whether that is with Microsoft technology, Java, or even android script to accept your Java or Android sign.
So, you're using Knockout and Javascript and you need to loop an array; are you tired of writing for loops with an if statement followed by break to exit the loop? I know I am, it must be a sign, so let's look at a better way to do it using ko.utils.arrayFirst.
Whether you are using the Bootstrap datepicker or the jQuery datepicker, they both use the same underlying JavaScript library. So with today's example, let's explore implementing the jQuery library with an added bonus of creating a Knockout custom binding. The end goal of this custom data bind is to create a form input field data bound not to the standard value binding, but instead to the custom one appropriately named: datepicker.
This example demonstrates how to create a custom ko.extender that will perform a JavaScript confirm prior to changing the value of your ko.observable. This snippet also contains a nice piece of additional functionality to only perform the confirm when a certain condition is met. For example, only show the confirm dialog when the observable is being changed from false to true.
This tutorial describes the best debugging technique to solve the dreaded "Uncaught ReferenceError: Unable to process binding" when developing with Knockout.js. When you are beginning your road with Knockout, it is very easy to make a mistake data bind an observable. This article demonstrates by example the most useful technique for debugging your data binding issue. Trial and error really helps you learn how Knockout works. This is the number one debug version to log and watch for comments in your ui and html website events whether it is in a form, document, or submit button. It works with any model and array or object value.
This tutorial demonstrates the ins-and-outs of creating a re-usable Knockout.js component with a very detailed and useful example. A Knockout component is a self-contained UI element that simplifies your code into bit size chunks allowing you to make re-usable elements with a component data bind. This knockout.js tutorial walks your through creating a component with html elements that will check/uncheck all checkboxes inside a computed observable array
In JavaScript, the `this` variable inside a function (like a computed observable or a KnockoutJS subscribe) function can be an extremely useful variable to access related properties to your observable. Learning the following behavior has opened many doors for me when leveraging the Knockout js framework. When declaring a computed observable, Knockout provides the ability for you to override what the `this` variable inside of your function's data that can be accessed. This is very similar Javascript code used for beginners instantiating the viewmodel with var self value as the initial input type of the function code on your website with the right details.
I have been using Knockout and Javascript afterRender template data bind function for years to hide a progress bar when the foreach loop completed. All of those years I have been using it incorrectly. Once I read Knockout's documentation about the foreach afterRender function, I realized that it is actually called after each render of the element inside the foreach. This is definitely not how I wanted this function to work. Let me show you my solution to accomplish applying the afterRender when the foreach function has completed.
Leveraging Bootstrap which provides many incredibly styled components for buttons, alert boxes, tables, forms, etc... regular radio buttons will be replaced by a button group (see screenshot below). Knockout.js will be used to create a custom data binding function that will make the group of buttons act like regular radio buttons (with a nicer look of course).

You have a list of elements - such as emails or any other list of data - and you want to provide the user a one-click button to select (or unselect) all items in the list. A nice added bonus, you want to automatically update the "global" checkbox indicating when all items are checked (and unchecking it when all items are not checked).
I can't believe 9 months has gone by since I came to an agreement on writing two books with O'Reilly Media! The first book was on Knockout.js which is a great framework that focuses on the Model-View-ViewModel (MVVM) architecture pattern. I finished the initial draft at the end of September 2014. During October and November, I multi-tasked by writing the second book while working through copy edits and multiple rounds of QC on the first book.
How does KnockoutJS Tutorial work?
KnockoutJS is different from a library like jQuery because it focuses on one particular aspect of a web page: binding data using observable variables, computed observables, and computed observable arrays. It doesn't do thing like AJAX requests; however, pairing Knockout.js with jQuery is an excellent way to perform an AJAX request then using the results to data bind to one of your view model variables. KnockoutJS will then detect, through a subscription, and tell the browser when to dynamically refresh the UI.
The library plays really nicely with server-side technologies like ASP.NET MVC and Web API. The Web API can be used to return JSON data via an AJAX call. With MVC, you can take your Model and convert it to a Knockout ViewModel in your Razor view. It is a seamless way to transition from MVC to MVVM.
If you are looking for a book about Knockout.js, I have written two books. The first Knockout.js book is focused on all aspects of Knockout that will take you from a beginner to expert in a few days. The second ASP.NET MVC 5 with Bootstrap and Knockout.js book demonstrates how to use MVC and Knockout.js that finishes with a full example on creating a shopping cart experience. It includes a very nice KnockoutJS component. Both books are available in PDF format.