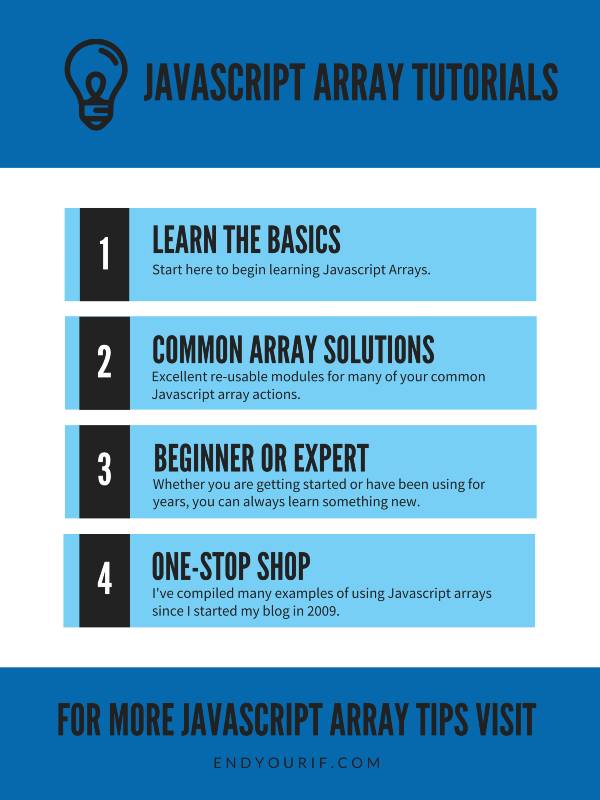
How do you create a Javascript Array?
In the following example there are two common ways to create empty array, one uses instantiation with the array constructor to create a new array instance and the other just initializes a new array object:
-
const fruits = new Array()
-
const fruits = []
Create an array with the array constructor
My personal preference is the second example simply because it's shorter and I guess the old school Javascript developer in me has never been used to having instantiate Javascript variables like I do in other languages like C#.
Example array elements
Of course you are not limited to only creating empty arrays, you can create an array and set values at the same time: var myArray = [1, 2, 3]. This array is just integers but you can also set it with strings: var myArray = ['a', 'b', 'c']. You can even create arrays with complex Javascript objects:
[code] var myArray = [{ company: 'EndYourIf', content: 'Javascript Arrays' }]; [/code]
As you will notice when adding new element to add multiple values you use a comma separated list with the string representing the elements of an array with an ordered collection.
Beginner Javascript Array Methods Examples
Below are a list of Javascript articles that will provide the essential tools to take you from a beginner to an expert with arrays by providing clear and concise demos with practical, real-world examples. Whether you are looking for beginner tutorials or advanced tutorials these examples will provide the essentials to become more proficient with everything relating to Javascript Arrays with the following example.
-
Group array objects by key with Javascript
-
Sort array of objects by any property value using Javascript
-
Merging two or more arrays and removing duplicates with Javascript
-
How to compare two or more arrays in Javascript
-
Using a forEach loop with JavaScript
-
Splitting an unordered list into multiple columns
-
Remove a specific element from an array
When possible, the following example attempts to discuss the execution speed based on the array size and number of array items. It doesn't matter whether there are two elements, three elements, no matter the element from an array it can be the first index, the current element there is always a way to access the correct element of the array.
The array length property will provide easy access to the array properties of an existing array whether it manipulates the original array when using square brackets that can span multiple lines as long as the array satisfies the array elements.
Javascript arrays and various array methods
As I continue adding new articles, I'll make sure I update this list to continue growing with more and more articles on JavaScript arrays. Whether they are to add elements, use the push method, updating the new length with the new keyword or even using a callback function that can be useful with the provided filtering function incombination with the provided testing function.
These examples will go over everything about array literal notation, handling two or more arrays, working with the array length to either access multiple elements, the first element, the last element or all the elements of an array.
With js functions I've created many new array methods that manipulate array elements with the length property, the index value. Sometimes the new array will access one or more elements of an array, or maybe just the first element and of course that would mean accessing the last element of an array or even just the third element but almost always accessing at least one element of the associative arrays.
Javascript array methods with square brackets
When you have an empty array accessing an array element with the original array will be difficult. Using that same object you can add new array elements with associative arrays via a comma separated list.
The array size can update the new length by using the push method which is a very important js functions. The push method will allow you to add elements by creating a new element. By adding another element you can update the original array with a second element which will increase the array size to two elements simply because you've added a new element.
Knockout.js Book
If you're looking how to use Javascript incombination with other programming languages and another framework that allows for creating rich, dynamic web pages I would highly suggest the book I wrote called Knockout.js - Building Dynamic Client-Side Applications where I demonstrate how to use an MVVM framework using jQuery as a strong companion library.
What do I do now that I've learned all about Javascript array element?
My blog has been around since 2009 and I've written 100s of articles where I've compiled common technologies into an organized, easy-to-follow examples. When you are familiar with JavaScript you can learn Knockout.js where I've written over 10 articles to get you started.
If you are interested in using JavaScript as a server-side language then I have also compiled a nice collection of Node.js tutorials that will get you started with using the Express package to create a web server using Node js as the server technology.